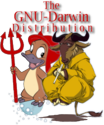
|
Generic Tcl Database Interface API
$Id: api.html,v 1.6 1998/07/23 01:54:36 tdarugar Exp proclus $
Your code must load the sql package using the normal method:
package require sql .
Alternatively you can simply load the shared object using something like:
load ./sql.so
The sql package adds only a single command to tcl:
sql . This command
takes several subcommands, as listed below. The subcommands
are always the first argument to the
sql command. The second
argument, if necessary, is the handle for a given connection.
Normal usage would be to create a connection via
connect , select a
database via selectdb ,
query or execute sql commands via
query and
exec , and
close the connection using
disconnect .
- connect <host> <userid> <password>
- Connect to the database. Returns the handle from that connection.
Takes two optional arguments, the userid and password.
set conn [sql connect]
set conn2 [sql connect host someuser somepassword]
- disconnect handle
- Disconnect the given connection.
sql disconnect $conn
- selectdb handle dbname
- Select the dbname database to be used on the given handle.
After doing a connect you must selectdb.
sql selectdb $conn mydatabase
- exec handle statement
- Execute a sql statement.
sql exec $conn "create table sample (x integer)"
- query handle statement
- Query the database for some results (generally a select
statement). Use with
fetchrow and endquery .
set query [sql query $conn "select * from sample where x > 3"]
- fetchrow handle
- Fetch the next row of results. Must be executed after a
query statement. The row is returned as a tcl
list. If there are no more rows available an empty string
is returned.
while {[set row [sql fetchrow $conn $query]] != ""} { puts $row }
Also see
sample.simple.txt and
sample.full.txt .
# Load the sql package:
package require sql
# Connect to the database
set conn [sql connect]
# select the database 'test' to be used.
sql selectdb $conn test
# Create a table and put some data in it:
sql exec $conn "create table junk (i integer, r real, s char(10))"
# Put some dummy data in:
for {set i 0} {$i < 10} {incr i} {
sql exec $conn "insert into junk values ($i, $i.01, 'xx $i xx')"
}
# Do a select and display the results
set query [sql query $conn "select * from junk where i > 3"]
while {[set row [sql fetchrow $conn $query]] != ""} {
puts "row = $row"
}
sql endquery $conn $query
sql exec $conn "drop table junk"
sql disconnect $conn
Results:
row = 4 4.0100 {xx 4 xx}
row = 5 5.0100 {xx 5 xx}
row = 6 6.0100 {xx 6 xx}
row = 7 7.0100 {xx 7 xx}
row = 8 8.0100 {xx 8 xx}
row = 9 9.0100 {xx 9 xx}
|