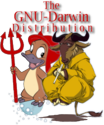
|
LOD - Level Of Detail group node which allows switching between children depending on distance from eye point.
Inheritance:
Public Methods-
LOD()
-
LOD(const LOD&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
-
META_Node(osg, LOD)
-
virtual void traverse(NodeVisitor& nv)
-
virtual bool addChild(Node* child)
-
virtual bool addChild(Node* child, float min, float max)
-
virtual bool removeChild(Node* child)
-
void setCenterMode(CenterMode mode)
-
CenterMode getCenterMode() const
-
inline void setCenter(const Vec3& center)
- Sets the object-space point which defines the center of the osg::LOD.
-
inline const Vec3& getCenter() const
- return the LOD center point.
-
void setRange(unsigned int childNo, float min, float max)
- Sets the min and max visible ranges of range of specifiec child.
-
inline float getMinRange(unsigned int childNo) const
- returns the min visible range for specified child
-
inline float getMaxRange(unsigned int childNo) const
- returns the max visible range for specified child
-
inline unsigned int getNumRanges() const
- returns the number of ranges currently set.
-
inline RangeList& getRangeList()
- return the list of MinMax ranges for each child
-
inline const RangeList& getRangeList() const
- return the list of MinMax ranges for each child
Public Members-
typedef std::pair<float,float> MinMaxPair
-
typedef std::vector<MinMaxPair> RangeList
-
enum CenterMode
Protected Fields-
CenterMode _centerMode
-
Vec3 _userDefinedCenter
-
RangeList _rangeList
Protected Methods-
virtual ~LOD()
Inherited from Group:
Public Methods-
virtual Group* asGroup()
-
virtual const Group* asGroup() const
-
virtual bool replaceChild( Node* origChild, Node* newChild )
-
inline unsigned int getNumChildren() const
-
virtual bool setChild( unsigned int i, Node* node )
-
inline Node* getChild( unsigned int i )
-
inline const Node* getChild( unsigned int i ) const
-
inline bool containsNode( const Node* node ) const
-
inline unsigned int getChildIndex( const Node* node ) const
Public Members-
typedef std::vector<ref_ptr<Node> > ChildList
Protected Fields-
ChildList _children
Protected Methods-
virtual bool computeBound() const
Inherited from Node:
Public Methods-
virtual Object* cloneType() const
-
virtual Object* clone(const CopyOp& copyop) const
-
virtual bool isSameKindAs(const Object* obj) const
-
virtual const char* libraryName() const
-
virtual const char* className() const
-
virtual Transform* asTransform()
-
virtual const Transform* asTransform() const
-
virtual void accept(NodeVisitor& nv)
-
virtual void ascend(NodeVisitor& nv)
-
inline void setName( const std::string& name )
-
inline void setName( const char* name )
-
inline const std::string& getName() const
-
inline const ParentList& getParents() const
-
inline ParentList getParents()
-
inline Group* getParent(unsigned int i)
-
inline const Group* getParent(unsigned int i) const
-
inline unsigned int getNumParents() const
-
void setUpdateCallback(NodeCallback* nc)
-
inline NodeCallback* getUpdateCallback()
-
inline const NodeCallback* getUpdateCallback() const
-
void setAppCallback(NodeCallback* nc)
-
inline NodeCallback* getAppCallback()
-
inline const NodeCallback* getAppCallback() const
-
inline unsigned int getNumChildrenRequiringUpdateTraversal() const
-
void setCullCallback(NodeCallback* nc)
-
inline NodeCallback* getCullCallback()
-
inline const NodeCallback* getCullCallback() const
-
void setCullingActive(bool active)
-
inline bool getCullingActive() const
-
inline unsigned int getNumChildrenWithCullingDisabled() const
-
inline bool isCullingActive() const
-
inline unsigned int getNumChildrenWithOccluderNodes() const
-
bool containsOccluderNodes() const
-
inline void setNodeMask(NodeMask nm)
-
inline NodeMask getNodeMask() const
-
inline const DescriptionList& getDescriptions() const
-
inline DescriptionList& getDescriptions()
-
inline const std::string& getDescription(unsigned int i) const
-
inline std::string& getDescription(unsigned int i)
-
inline unsigned int getNumDescriptions() const
-
void addDescription(const std::string& desc)
-
inline void setStateSet(osg::StateSet* dstate)
-
osg::StateSet* getOrCreateStateSet()
-
inline osg::StateSet* getStateSet()
-
inline const osg::StateSet* getStateSet() const
-
inline const BoundingSphere& getBound() const
-
void dirtyBound()
Public Members-
typedef std::vector<Group*> ParentList
-
typedef unsigned int NodeMask
-
typedef std::vector<std::string> DescriptionList
Protected Fields-
mutable BoundingSphere _bsphere
-
mutable bool _bsphere_computed
-
std::string _name
-
ParentList _parents
-
ref_ptr<NodeCallback> _updateCallback
-
unsigned int _numChildrenRequiringUpdateTraversal
-
ref_ptr<NodeCallback> _cullCallback
-
bool _cullingActive
-
unsigned int _numChildrenWithCullingDisabled
-
unsigned int _numChildrenWithOccluderNodes
-
NodeMask _nodeMask
-
DescriptionList _descriptions
-
ref_ptr<StateSet> _stateset
Protected Methods-
void addParent(osg::Group* node)
-
void removeParent(osg::Group* node)
-
void setNumChildrenRequiringUpdateTraversal(unsigned int num)
-
void setNumChildrenWithCullingDisabled(unsigned int num)
-
void setNumChildrenWithOccluderNodes(unsigned int num)
Inherited from Object:
Public Methods-
inline void setDataVariance(DataVariance dv)
-
inline DataVariance getDataVariance() const
-
inline void setUserData(Referenced* obj)
-
inline Referenced* getUserData()
-
inline const Referenced* getUserData() const
Public Members-
enum DataVariance
Protected Fields-
DataVariance _dataVariance
-
ref_ptr<Referenced> _userData
Public Methods-
inline Referenced& operator = (Referenced&)
-
static void setDeleteHandler(DeleteHandler* handler)
-
static DeleteHandler* getDeleteHandler()
-
inline void ref() const
-
inline void unref_nodelete() const
-
inline int referenceCount() const
-
inline void unref() const
Protected Fields-
mutable int _refCount
Documentation
LOD - Level Of Detail group node which allows switching between children
depending on distance from eye point.
Typical uses are for load balancing - objects further away from
the eye point are rendered at a lower level of detail, and at times
of high stress on the graphics pipeline lower levels of detail can
also be chosen.
The children are ordered from most detailed (for close up views) to the least
(see from a distance), and a set of ranges are used to decide which LOD is used
at different view distances, the criteria used is child 'i' is used when
range[i]
LOD()
LOD(const LOD&, const CopyOp& copyop=CopyOp::SHALLOW_COPY)
- Copy constructor using CopyOp to manage deep vs shallow copy
META_Node(osg, LOD)
virtual void traverse(NodeVisitor& nv)
virtual bool addChild(Node* child)
virtual bool addChild(Node* child, float min, float max)
virtual bool removeChild(Node* child)
typedef std::pair<float,float> MinMaxPair
typedef std::vector<MinMaxPair> RangeList
enum CenterMode
USE_BOUNDING_SPHERE_CENTER
USER_DEFINED_CENTER
void setCenterMode(CenterMode mode)
CenterMode getCenterMode() const
inline void setCenter(const Vec3& center)
- Sets the object-space point which defines the center of the osg::LOD.
center is affected by any transforms in the hierarchy above the osg::LOD.
inline const Vec3& getCenter() const
- return the LOD center point.
void setRange(unsigned int childNo, float min, float max)
- Sets the min and max visible ranges of range of specifiec child.
Values are floating point distance specified in local objects coordinates.
inline float getMinRange(unsigned int childNo) const
- returns the min visible range for specified child
inline float getMaxRange(unsigned int childNo) const
- returns the max visible range for specified child
inline unsigned int getNumRanges() const
- returns the number of ranges currently set.
An LOD which has been fully set up will have getNumChildren()==getNumRanges().
inline RangeList& getRangeList()
- return the list of MinMax ranges for each child
inline const RangeList& getRangeList() const
- return the list of MinMax ranges for each child
virtual ~LOD()
CenterMode _centerMode
Vec3 _userDefinedCenter
RangeList _rangeList
- Direct child classes:
- Impostor
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|